一个可以高亮纽约时段的工具
从国外一网站上看到的,大家用用看:
//+------------------------------------------------------------------+
//| ShadeNY_v5.mq4 |
//| Copyright © 2006, sx ted |
//| Purpose: shade New York or other sessions for chart time frames |
//| M1 to H4 (at a push). |
//| Notes..: To change chart time frame from M5 to M15 for example, |
//| click on M15, M5 and M15 again to force a chart refresh.|
//| Modify the values of MAX_DAYS_TO_SHADE, SESSION_OPEN_HH,|
//| SESSION_OPEN_MM, SESSION_CLOSE_HH and SESSION_CLOSE_MM |
//| to suit ***** THE LOCAL TIMES AT THE EXCHANGE *****. |
//| The values for SERVER_TIME_ZONE and EXCHANGE_TIME_ZONE |
//| are to be edited for DST when applicable. |
//| version: 2 - enhanced for speed but with MT4 beeing so fast no |
//| difference will be noticed, all the sessions are |
//| shaded in the init(), last session if it is current |
//| is widened in the start() in lieu of repainting all.|
//| 3 - 2006.03.22 added "SetImmediacyON" input parameter |
//| which forces shading after first tick is received |
//| in the new session. Corrected case where bar is not |
//| complete and session starts, and case when session |
//| covers two days when in Moscow (GMT+3 and greater). |
//| 4 - 2006.03.29 corrected for not taking into account the|
//| difference between Local time and Server time. |
//| 5 - 2006.04.03 corrected for Server time change, open |
//| and close times of exchange are now expressed in |
//| local times of the exchange. |
//| Added new SERVER_TIME_ZONE and EXCHANGE_TIME_ZONE in|
//| the #define section. Finally learnt about GMT time! |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2006, sx ted"
#property link ""
#property indicator_chart_window
//---- input parameters
extern color ShadeColor=Yellow;
extern bool SetImmediacyON=true; // if set ON forces shading after first tick in new session
#define MAX_DAYS_TO_SHADE 5 // maximum number of days back from last chart date to be shaded
#define SERVER_TIME_ZONE +2 // MetaTrader server time zone, compare CurTime() with Greenwich GMT
#define EXCHANGE_TIME_ZONE -4 // Exchange time zone, example New York -4 GMT
#define SESSION_OPEN_HH 09 // session open hour (in local time at the exchange)
#define SESSION_OPEN_MM 30 // session open minutes (in local time at the exchange)
#define SESSION_CLOSE_HH 16 // session close hour (in local time at the exchange)
#define SESSION_CLOSE_MM 05 // session close minutes (in local time at the exchange)
//---- global variables to program
string obj; // array of object names
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int init()
{
if(Period() > PERIOD_H4) return(0); // no shading required
// comment out or delete the following line when no longer required
Alert( "ServerTime at ", ServerAddress(), " is ", TimeToStr( CurTime(),TIME_MINUTES ), "\nAdjust #define SERVER_TIME_ZONE if required" );
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
int deinit()
{
for(int i=0; i < ArraySize(obj); i++)
{
if(ObjectFind(obj) > -1) ObjectDelete(obj); // tidy up
}
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
if(Period() > PERIOD_H4) return(0); // no shading required
int i, iCount, iAdjust, // counters
iStart, iEnd; // x co-ordinates of object (index)
double dLow, dHigh; // y co-ordinates of object
string sName; // name for the object
int iSessionDuration; // session duration in seconds
bool ok;
// adjust for difference between Server time and Local time at the exchange (in minutes)
iAdjust=(SERVER_TIME_ZONE - EXCHANGE_TIME_ZONE) * 60;
// determine open time of first bar in the session matching chart time frame in format " hh:mi"
iStart=SESSION_OPEN_HH*60+SESSION_OPEN_MM+iAdjust;
if(iStart > 24*60) iStart-=24*60;
string sStart=" "+TimeToStr(StrToTime("2006.3.22")+(MathFloor(iStart/Period()) * Period() ) * 60, TIME_MINUTES);
// calculate session duration in seconds, check if session covers two days (cater for GMT+3 and above)
if(SESSION_OPEN_HH*60+SESSION_OPEN_MM >= SESSION_CLOSE_HH*60+SESSION_CLOSE_MM) iSessionDuration = ((23-SESSION_OPEN_HH+SESSION_CLOSE_HH)*60+59-SESSION_OPEN_MM+SESSION_CLOSE_MM+1)*60;
else iSessionDuration = ( (SESSION_CLOSE_HH*60+SESSION_CLOSE_MM) - (SESSION_OPEN_HH*60+SESSION_OPEN_MM) )*60;
// round session duration to suit an open time of chart time frame
datetime tStart =StrToTime(TimeToStr(Time[0], TIME_DATE)+sStart);
datetime tEnd =StrToTime(TimeToStr(Time[0], TIME_DATE)+" "+DoubleToStr(SESSION_OPEN_HH ,0)+":"+DoubleToStr(SESSION_OPEN_MM ,0))+iSessionDuration+iAdjust*60;
iSessionDuration=MathCeil((tEnd-tStart)/Period())*Period();
// clear previous objects
for(i=0; i < ArraySize(obj); i++)
{
if(ObjectFind(obj) > -1) ObjectDelete(obj);
}
for(i=MAX_DAYS_TO_SHADE; i >= 0; i--)
{
tStart=StrToTime(TimeToStr(iTime(NULL, PERIOD_D1, i), TIME_DATE)+sStart);
tEnd =tStart+iSessionDuration;
iStart=iBarShift(NULL, 0, tStart, false);
ok=(iStart > 0 && iStart < Bars-1 && TimeDayOfYear(tStart) == TimeDayOfYear(Time[iStart]));
if(!ok && SetImmediacyON && CurTime() >= tStart && CurTime() < tEnd)
{
ok=true;
iStart=1;
}
if(ok)
{
iEnd=iBarShift(NULL, 0, tEnd, false);
if(iEnd >= Bars-1) iEnd=0; // end not found, therefore current session
while(iEnd < iStart && Time[iEnd] > tEnd) iEnd++; // cater for earlier close on Friday
dLow =Low[Lowest(NULL,0,MODE_LOW,iStart-iEnd,iEnd)];
dHigh=High[Highest(NULL,0,MODE_HIGH,iStart-iEnd,iEnd)];
sName="Session_"+TimeToStr(iTime(NULL, PERIOD_D1, i), TIME_DATE);
ObjectCreate(sName,OBJ_RECTANGLE,0,Time[iStart],dLow-Point,Time[iEnd],dHigh+Point);
ObjectSet(sName,OBJPROP_COLOR,ShadeColor);
// keep track of object names for tidying up upon exit
iCount=ArraySize(obj);
ArrayResize(obj, iCount+1);
obj[iCount]=sName;
}
}
return(0);
}
//+------------------------------------------------------------------+
[ 本帖最后由 老正 于 2006-6-12 20:55 编辑 ]
shadeny_v3.gif
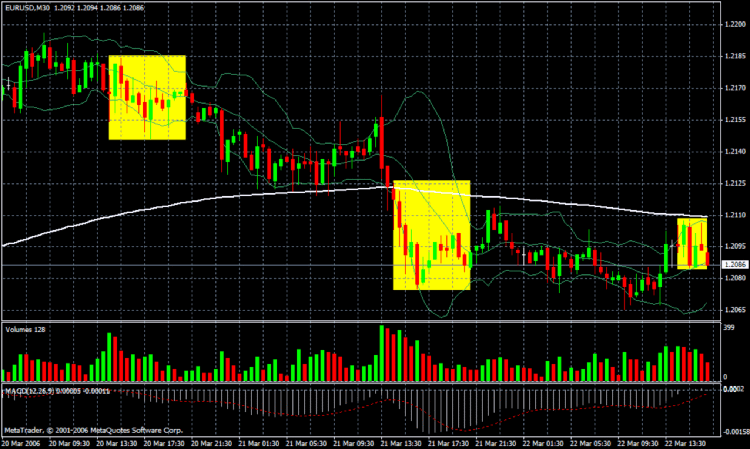
发表于:2006-06-11 05:57只看该作者
2楼
如何使用?
韬客社区www.talkfx.co
发表于:2006-06-12 05:20只看该作者
3楼
报错呀!!
韬客社区www.talkfx.co
发表于:2006-06-12 12:56只看该作者
4楼
修改了下代碼的顯示
应该是可以用了吧
遇到矛盾 先站在对方的立场上想想问题,先试着去理解别人
● 如何使用WinMTR查询平台连接流畅度
发表于:2006-06-13 07:31只看该作者
5楼
请教如何改为伦敦时段
韬客社区www.talkfx.co
发表于:2006-06-14 05:32只看该作者
6楼
要是能将日本时段---德国时段---美国时段用3色显示出来就完美了
韬客外汇论坛TALKFOREX.COM
7楼
各个市场的具体交易时间是怎么样?
我来改一改试试,应该可以的.
它原先设定纽约是从9:30到4:05,纽约时间
韬客社区www.talkfx.co
发表于:2006-06-19 02:31只看该作者
8楼
原帖由 bavttt 于 2006-6-17 00:15 发表 各个市场的具体交易时间是怎么样? 我来改一改试试,应该可以的. 它原先设定纽约是从9:30到4:05,纽约时间
发表于:2006-06-19 07:16只看该作者
9楼
可以高亮两个自定义时段的指标:
//+------------------------------------------------------------------+
//| i-ParamonWorkTime.mq4 |
//| http://www.kimiv.ru |
//| |
//| 23.11.2005 |
//+------------------------------------------------------------------+
#property copyright "aka KimIV"
#property link "http://www.kimiv.ru"
#property indicator_chart_window
//------- -------------------------------
extern int NumberOfDays = 50;
extern string Begin_1 = "07:00";
extern string End_1 = "11:00";
extern color Color_1 = BlanchedAlmond;
extern string Begin_2 = "13:00";
extern string End_2 = "17:00";
extern color Color_2 = Lavender;
extern string Begin_3 = "19:00";
extern string End_3 = "23:00";
extern color Color_3 = MistyRose;
extern bool HighRange = False;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
void init() {
DeleteObjects();
for (int i=0; i5) dt=decDateTradeDay(dt);
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void DrawObjects(datetime dt, string no, string tb, string te) {
datetime t1, t2;
double p1, p2;
int b1, b2;
t1=StrToTime(TimeToStr(dt, TIME_DATE)+" "+tb);
t2=StrToTime(TimeToStr(dt, TIME_DATE)+" "+te);
b1=iBarShift(NULL, 0, t1);
b2=iBarShift(NULL, 0, t2);
p1=High[Highest(NULL, 0, MODE_HIGH, b1-b2, b2)];
p2=Low [Lowest (NULL, 0, MODE_LOW , b1-b2, b2)];
if (!HighRange) {p1=0; p2=2*p2;}
ObjectSet(no, OBJPROP_TIME1 , t1);
ObjectSet(no, OBJPROP_PRICE1, p1);
ObjectSet(no, OBJPROP_TIME2 , t2);
ObjectSet(no, OBJPROP_PRICE2, p2);
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
datetime decDateTradeDay (datetime dt) {
int ty=TimeYear(dt);
int tm=TimeMonth(dt);
int td=TimeDay(dt);
int th=TimeHour(dt);
int ti=TimeMinute(dt);
td--;
if (td==0) {
tm--;
if (tm==0) {
ty--;
tm=12;
}
if (tm==1 || tm==3 || tm==5 || tm==7 || tm==8 || tm==10 || tm==12) td=31;
if (tm==2) if (MathMod(ty, 4)==0) td=29; else td=28;
if (tm==4 || tm==6 || tm==9 || tm==11) td=30;
}
return(StrToTime(ty+"."+tm+"."+td+" "+th+":"+ti));
}
//+------------------------------------------------------------------+
韬客社区www.talkfx.co
发表于:2006-06-20 09:57只看该作者
11楼
谢了.
巨大的盈利来源于坐等,而不是思考.
发表于:2006-06-21 10:18只看该作者
12楼
原帖由 GBP 于 2006-6-19 15:16 发表 可以高亮两个自定义时段的指标: //+------------------------------------------------------------------+ //| i-ParamonWorkTime.mq4 | //| ...

韬客社区www.talkfx.co